Connect social providers to user accounts
2 April 2024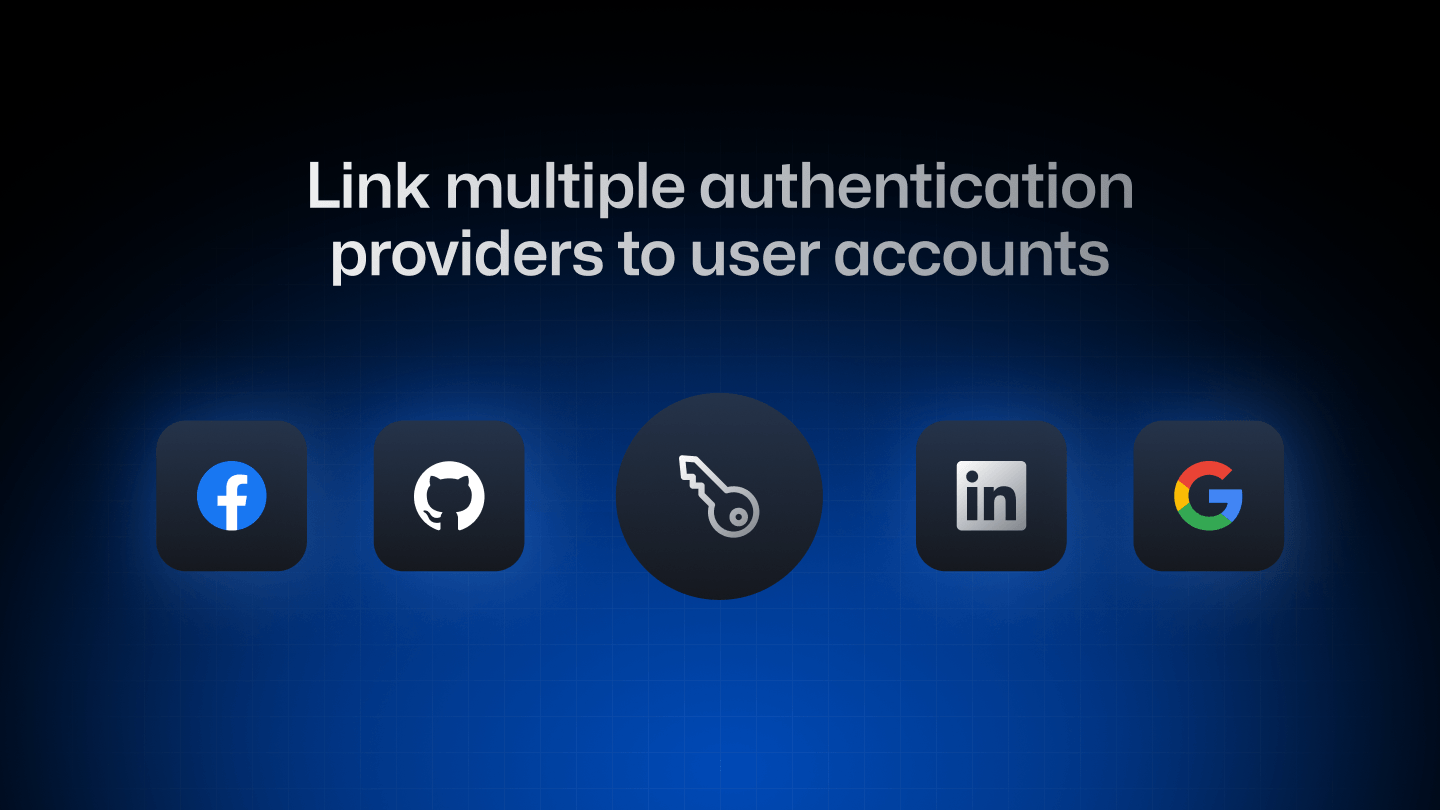
You can now allow existing users to connect different social logins to their account and use them alongside any other authentication mechanism, even if the email doesn't match the one they signed up with on your app. Users maintain the same user ID regardless of the authentication method they use. For instance, a user who initially signed in with a password can effortlessly link a GitHub account and utilize both for future logins. With just a few simple steps, you can seamlessly integrate this feature into your app.
1. Enable the authentication provider
The first thing you have to do, is enable the authentication provider you want your users to be able to connect to. For example, in the screenshot below we enable sign-in with GitHub which you can find when you click on Sign-In Methods.
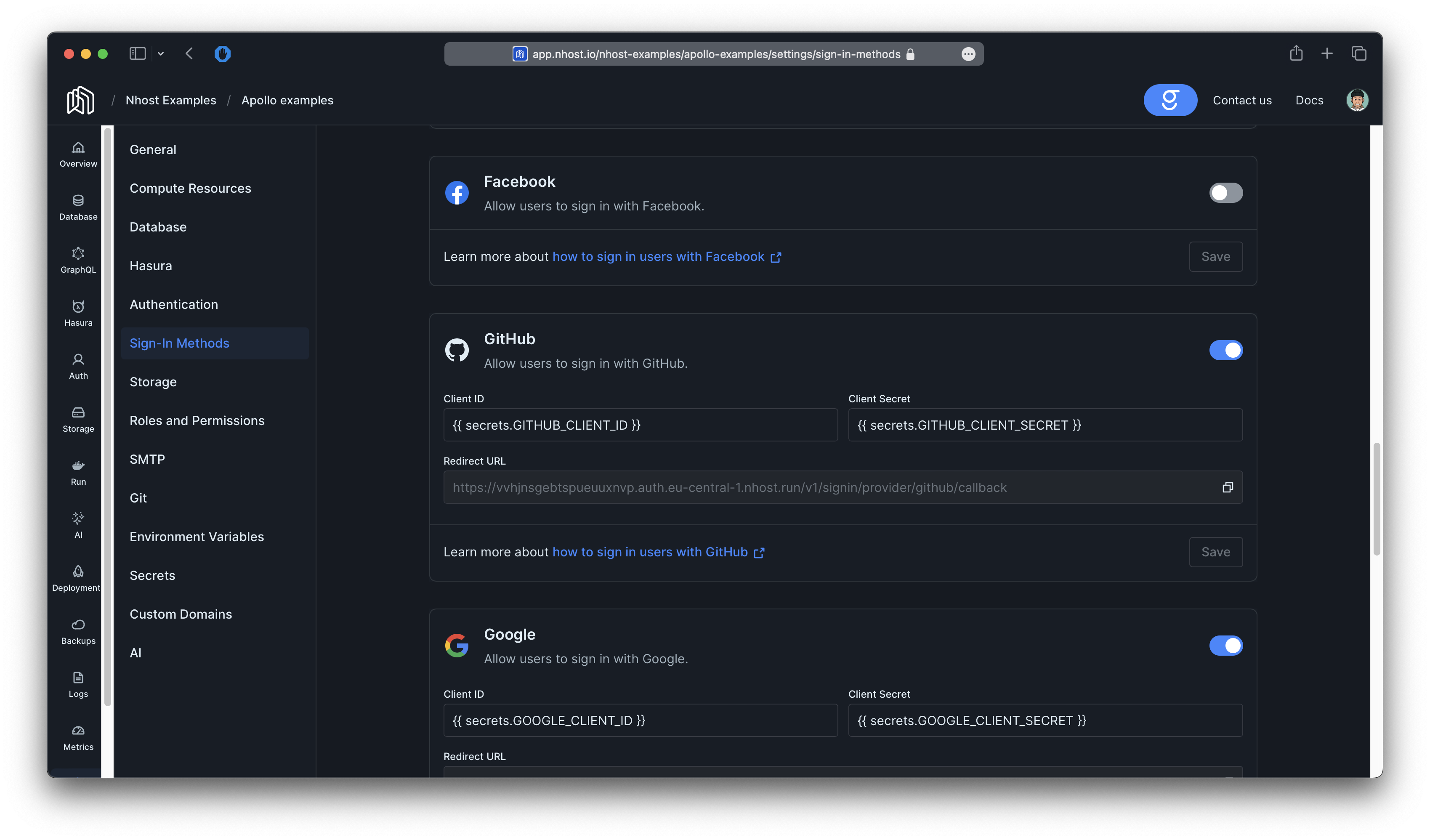
2. Generate the link from our SDK's
With the provider configured, you can use our SDK to redirect the user to hasura-auth to communicate with the authentication provider.
The following is an example of how to do that with React.
_15import React from 'react'_15import { useProviderLink } from '@nhost/react'_15_15export const Profile: React.FC = () => {_15 const { github } = useProviderLink({_15 connect: true,_15 redirectTo: `${window.location.origin}/profile`,_15 })_15_15 return (_15 <div>_15 <a href={github}>Connect with GitHub</a>_15 </div>_15 )_15}
Notice we've added a new paramater that you must pass to the useProviderLink
hook to generate the right link.
_10const { github } = useProviderLink({_10+ connect: true,_10 redirectTo: `${window.location.origin}/profile`,_10})
3. Verify that the provider was added to the user's account
To verify that the provider was linked to the user's account we just need to query the user_providers
table.
_16const { error, data } = await nhost.graphql.request(_16 gql`_16 query getAuthUserProviders {_16 authUserProviders {_16 id_16 providerId_16 }_16 }_16 `,_16)_16_16console.log({_16 isGitHubConnected: data?.authUserProviders?.some(_16 (item) => item.providerId === 'github',_16 ),_16})
4. Putting it all together
Here's what an example page in React looks like after following the steps above. Now we just need to check if the user has already connected their GitHub account and only show the link otherwise.
_46import React from 'react'_46import { useProviderLink, useNhostClient } from '@nhost/react'_46_46export const Profile: React.FC = () => {_46 const userId = useUserId()_46 const nhost = useNhostClient()_46 const [isGitHubConnected, setIsGitHubConnected] = useState(false)_46_46 const { github } = useProviderLink({_46 connect: true,_46 redirectTo: `${window.location.origin}/profile`,_46 })_46_46 useEffect(() => {_46 const getSecurityKeys = async () => {_46 const { error, data } = await nhost.graphql.request(_46 gql`_46 query getAuthUserProviders {_46 authUserProviders {_46 id_46 providerId_46 }_46 }_46 `,_46 )_46_46 if (!error) {_46 setIsGitHubConnected(_46 data?.authUserProviders?.some((item) => item.providerId === 'github'),_46 )_46 }_46 }_46_46 getSecurityKeys()_46 }, [nhost])_46_46 return (_46 <div>_46 {isGitHubConnected ? (_46 <span>GitHub connected</span>_46 ) : (_46 <a href={github}>Connect with GitHub</a>_46 )}_46 </div>_46 )_46}
Wrap up
By following the steps outlined above, you can enable your users to connect their account with an authentication provider. This proves to be incredibly valuable when integrating features into your app that depend on using the authentication provider's APIs. With this feature, you can alleviate concerns about users having signed up with a different email previously.